공업/방업 수치가 동일한 저글링은 같은 저글링으로 가정하고,중복 제거하여 1기로 취급하도록 구현 |
1. 저글링 클래스
package prac01;
public class Zergling {
// 필드
String name; // 유닛명
int attackUp; // 공업 단계(0~3)
int armorUp; // 방업 단계(0~3)
// 생성자
public Zergling(String name, int attackUp, int armorUp) {
this.name = name;
this.attackUp = attackUp;
this.armorUp = armorUp;
}
@Override
public String toString() {
return "Zergling [name=" + name + ", attackUp=" + attackUp + ", armorUp=" + armorUp + "]";
}
}
2.1. HashSet을 이용한 중복제거 실행 클래스
package prac01;
import java.util.HashSet;
import java.util.Set;
public class ZerglingMain {
public static void main(String[] args) {
Set<Zergling> set = new HashSet<>();
Zergling z1 = new Zergling("Zergling", 0, 0); // 노업 저글링
Zergling z2 = new Zergling("Zergling", 0, 0); // 노업 저글링
Zergling z3 = new Zergling("Zergling", 0, 0); // 노업 저글링
Zergling z4 = new Zergling("Zergling", 3, 0); // 공3업 저글링
Zergling z5 = new Zergling("Zergling", 3, 3); // 풀업 저글링
Zergling z6 = new Zergling("Zergling", 3, 3); // 풀업 저글링
Zergling z7 = new Zergling("Zergling", 1, 1); // 공방1업 저글링
set.add(z1);
set.add(z2);
set.add(z3);
set.add(z4);
set.add(z5);
set.add(z6);
set.add(z7);
System.out.println("set.size(): " + set.size());
for (Zergling z : set) {
System.out.println(z.hashCode());
System.out.println(z);
}
}
}
2.2. 실행결과
set.size(): 7
2046562095
Zergling [name=Zergling, attackUp=0, armorUp=0]
932172204
Zergling [name=Zergling, attackUp=3, armorUp=3]
1225358173
Zergling [name=Zergling, attackUp=1, armorUp=1]
1342443276
Zergling [name=Zergling, attackUp=0, armorUp=0]
1587487668
Zergling [name=Zergling, attackUp=3, armorUp=0]
1199823423
Zergling [name=Zergling, attackUp=3, armorUp=3]
769287236
Zergling [name=Zergling, attackUp=0, armorUp=0]
※ 중복제거 실패
3.1. 저글링 클래스 보완(hashCode, equals 메소드 재정의)
package prac01;
public class Zergling {
// 필드
String name; // 유닛명
int attackUp; // 공업 단계(0~3)
int armorUp; // 방업 단계(0~3)
// 생성자
public Zergling(String name, int attackUp, int armorUp) {
this.name = name;
this.attackUp = attackUp;
this.armorUp = armorUp;
}
@Override
public int hashCode() {
return this.name.hashCode() + this.attackUp + this.armorUp;
}
@Override
public boolean equals(Object obj) {
if (obj instanceof Zergling) {
Zergling tmp = (Zergling)obj;
if (this.attackUp == tmp.attackUp && this.armorUp == tmp.armorUp) {
return true;
}
}
return false;
}
@Override
public String toString() {
return "Zergling [name=" + name + ", attackUp=" + attackUp + ", armorUp=" + armorUp + "]";
}
}
3.2. 저글링 클래스 보완 후 재실행 결과
set.size(): 4
744866486
Zergling [name=Zergling, attackUp=0, armorUp=0]
744866492
Zergling [name=Zergling, attackUp=3, armorUp=3]
744866489
Zergling [name=Zergling, attackUp=3, armorUp=0]
744866488
Zergling [name=Zergling, attackUp=1, armorUp=1]
※ 중복제거 성공 (공업, 방업 수치가 동일한 저글링은 같은 개체로 취급)
참조
marobiana.tistory.com/100
superfelix.tistory.com/61
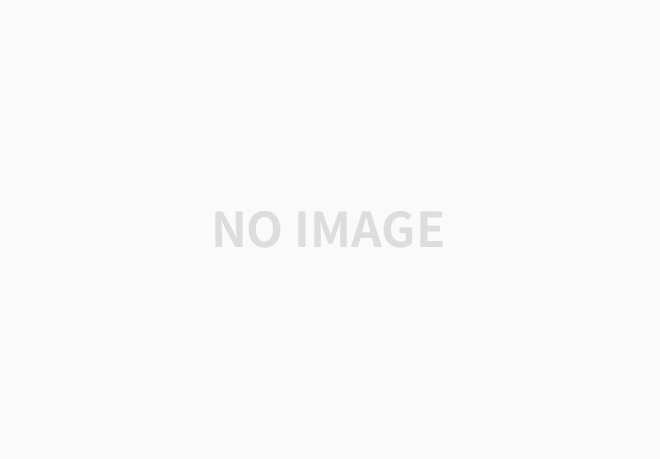
'Java' 카테고리의 다른 글
[Java] Jaxen을 이용한 XML 파싱 (0) | 2023.03.26 |
---|---|
[Java] Mybatis Mapper(xml) 파일에서 테이블명 및 뷰명만 추출 (0) | 2021.02.16 |